Quickstart
Installation
Download and install the extension from the Visual Studio Marketplace: https://marketplace.visualstudio.com/items?itemName=vi-sit.twizzar-vs22.
After the installation, restart Visual Studio. The extension should now be available from the Extensions menu:
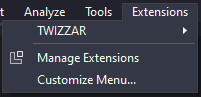
Create your first test with TWIZZAR
1. Create a new Unit Test
After installing the extension, you are ready to create your first test with TWIZZAR. To do this, right-click on the method you want to test and select "Create Unit Test" from the context menu. You also can use the shortcut Ctrl + Alt + N, Ctrl + Alt + N.
Note
To use the create command, the editor cursor must be on the method signature or in the method block.
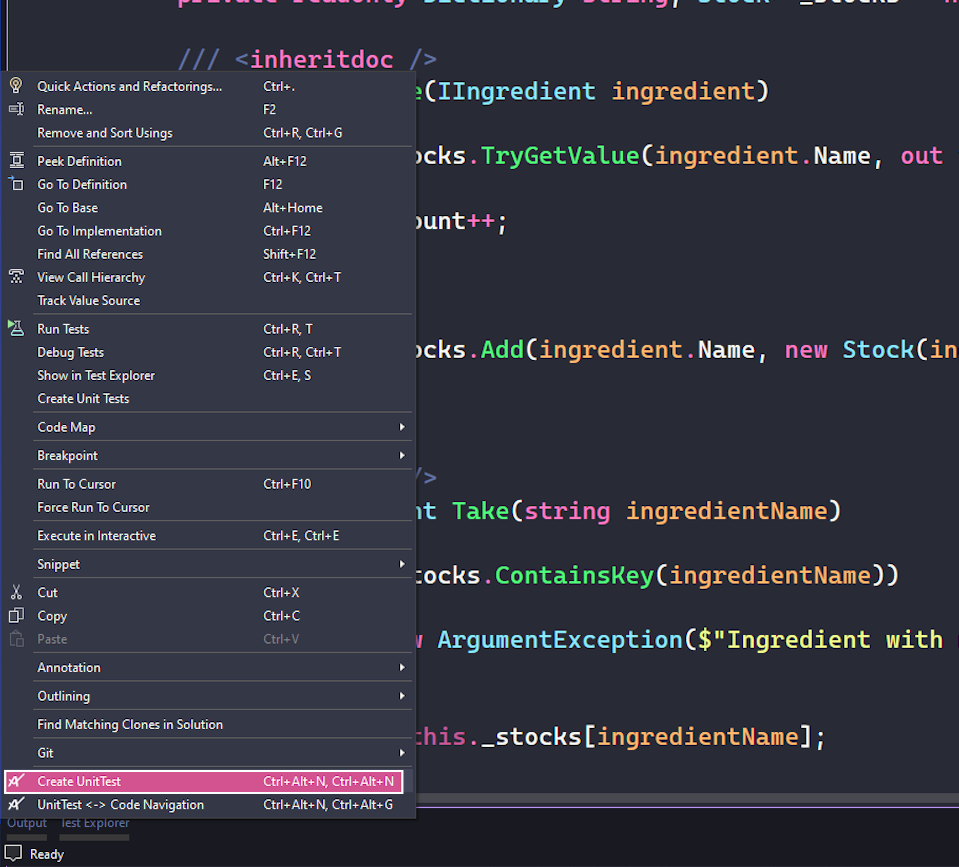
TWIZZAR will now create a test class for you and insert the necessary code to create a test with TWIZZAR. If TWIZZAR cannot find the test project, you will be asked if TWIZZAR should create one for you.
Note
To customize the test generation process please see Key concepts > Test creation > Configuration.
using NUnit.Framework;
using TWIZZAR.Fixture;
using PotionDeliveryService.Interfaces;
using PotionDeliveryService;
using System;
namespace PotionDeliveryService.Tests
{
[TestFixture]
public class StorageTests
{
[Test]
[TestSource(nameof(Storage.Store))]
public void Store_Scenario_ExpectedBehavior()
{
// Arrange
var sut = new ItemBuilder<Storage>().Build();
var ingredient = new ItemBuilder<IIngredient>().Build();
// Act
sut.Store(ingredient);
// Assert
Assert.Fail();
}
}
}
using NUnit.Framework;
using TWIZZAR.Fixture;
using PotionDeliveryService.Interfaces;
using PotionDeliveryService;
using System;
namespace PotionDeliveryService.Tests
{
[TestFixture]
public class StorageTests
{
[Test]
[TestSource(nameof(Storage.Store))]
public void Store_Scenario_ExpectedBehavior()
{
// Arrange
var sut = new ItemBuilder<Storage>().Build();
var ingredient = new ItemBuilder<IIngredient>().Build();
// Act
sut.Store(ingredient);
// Assert
Assert.Fail();
}
}
}
TWIZZAR creates an arrange block (Line 17-18) for you, the sut
on line 17 is the System Under Test, this is the class which is tested. The ingredient
on line 18 is the parameter of the method which is tested. They all use the ItemBuilder
to create a new instance of the type. The ItemBuilder
is a class of the TWIZZAR API. It is used to create a new instance of a type and configure it. For more information about the ItemBuilder
see Item Builder.
In the act block (Line 21), the method which is tested is called with the parameters created in the arrange block.
The assert block (Line 24) only has a fail condition, this is because TWIZZAR does not know what should be asserted.
2. Rename the test
TWIZZAR generates a placeholder name for the unit test, this name should be changed to a meaningful name.
⋯
[Test]
[TestSource(nameof(Storage.Store))]
public void Store_OnStore_IngredientIsAvailable()
⋯
⋯
[Test]
[TestSource(nameof(Storage.Store))]
public void Store_OnStore_IngredientIsAvailable()
⋯
3. Set up the System Under Test
The ItemBuilder
class of TWIZZAR uses the builder pattern to set up an instance. It can be configured with the TWIZZAR UI or by code.
Note
The UI and the code both use the dependency tree to select members to set up. To learn more about the dependency tree see ItemBuilder > Dependency Tree.
TWIZZAR UI
To open the TWIZZAR UI use the arrow behind the ItemBuilder
or use the TWIZZAR open/close shortcut (Ctrl + Alt + N, Ctrl + Alt + V). Configure the member by entering a value in the input field.

TWIZZAR will create a builder class inheriting from new ItemBuilder<IIngredient>()
. Rename this class to something meaningful, for example, WaterBuilder
.
Configure by code
To configure the ItemBuilder
by code, use the With
method. The With
method accepts a function that returns a MemberConfig
. To create this MemberConfig
, TWIZZAR provides a path class as a parameter to the function to select and then configure a member.
var ingredient = new ItemBuilder<IIngredient>()
.With(p => p.Name.Value("Water"))
.Build();
var ingredient = new ItemBuilder<IIngredient>()
.With(p => p.Name.Value("Water"))
.Build();
4. Assert the test
To finish the test we can now write the assertion.
⋯
// Assert
Assert.IsTrue(sut.CheckAvailable(ingredient.Name));
⋯
// Assert
Assert.IsTrue(sut.CheckAvailable(ingredient.Name));
Note
TWIZZAR provides a Verify
method for method or property verification. For more information see Verify.
5. Run the test
The test can now be run with the standard Visual Studio test runner or any other test runner of your choice. This was all needed for this test to run successfully.
Examples
An example project can be found under examples/PotionDeliveryService, this is a good starting point to get familiar with TWIZZAR.